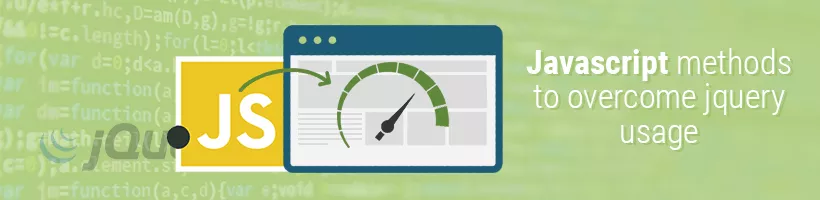
16 Nov 2017
Fab 15 JavaScript methods to overcome jQuery usage
Every business must make sure that their websites work fast on all devices. The users are extremely sensitive to web performance, even the smallest change in the website load time alters traffic noticeably. The website should be spontaneously reactive and engage visitors quickly for every action visitor performs.
Most of the developers use certain javascript libraries like jQuery, which make it easier for them to develop frontend functionalities. But take a moment to consider if you actually need jQuery as a dependency. If you're only targeting more modern browsers, you might not need anything more than what the browser ships with, post-IE8, all browsers are pretty easy to deal with on their own.
The major disadvantage of using jQuery on the website is the size of jQuery, which is 270 kB, and will be loaded on all the pages of your website. You may load the 30 kB minified version from a CDN but the browser will halt processing and parse the code on every page before doing anything else.
So let us see how we can avoid using jQuery library on our website, by using the equivalent native javascript methods.
1. querySelector
jQuery Code
* var paragraph = $("p");
Equivalent JavaScript Code
* var paragraph = document.querySelectorAll("p");
Other DOM selector methods
document.querySelector("p") /* fetches the first paragraph. */
document.getElementsByClassName(class) /* fetches all the nodes having this class name */
2. append / appendChild
jQuery Code
$("div").append("more content");
Equivalent JavaScript Code
var p = document.createElement("p"); p.appendChild(document.createTextNode("more content")); document.querySelector("div").appendChild(p);
3. innerHTML
jQuery Code
$("div").html("more content");
Equivalent JavaScript Code
document.querySelector("div").innerHTML = "more content";
4. removeChild
jQuery Code
$("#container").remove();
Equivalent JavaScript Code
var c = document.getElementById("container"); c.parentNode.removeChild(c);
5. XMLHttpRequest
jQuery Code
$.ajax({ type: 'GET', url: '/my/url', success: function(resp) { }, error: function() { } });
Equivalent JavaScript Code
var request = new XMLHttpRequest(); request.open('GET', '/my/url', true); request.onload = function() { if (request.status >= 200 && request.status < 400) { // Success! var resp = request.responseText; var resp = request.responseText; } else { // We reached our target server, but it returned an error } }; request.onerror = function() { // There was a connection error of some sort }; request.send();
6. style
jQuery Code
$("#container").css("display","none");
Equivalent JavaScript Code
var c = document.getElementById("container"); c.style.display = 'none'; 7. className / classList.add
jQuery Code
$("#container").addClass(className);
Equivalent JavaScript Code
var c = document.getElementById("container"); if (c.classList) c.classList.add(className); else c.className += ' ' + className;
8. insertAdjacentHTML
jQuery Code
$("#container").after(htmlString);
Equivalent JavaScript Code
var c = document.getElementById("container"); c.insertAdjacentHTML('afterend', htmlString);
9. insertAdjacentHTML
jQuery Code
$("#container").before(htmlString);
Equivalent JavaScript Code
var c = document.getElementById("container"); c..insertAdjacentHTML('beforebegin', htmlString);
10. children
jQuery Code
$("#container").children();
Equivalent JavaScript Code
var c = document.getElementById("container"); c.children
11. forEach
jQuery Code
$.each(array, function(i, item){ });
Equivalent JavaScript Code
array.forEach(function(item, i){ });
12. getAttribute
jQuery Code
$(el).attr('tabindex');
Equivalent JavaScript Code
var c = document.getElementById("container"); c..getAttribute('tabindex');
13. parentNode
jQuery Code
$(el).parent();
Equivalent JavaScript Code
el.parentNode
14. setAttribute
jQuery Code
$(el).attr('tabindex', 3);
Equivalent JavaScript Code
el.setAttribute('tabindex', 3);
15. addEventListener
jQuery Code
$("p").click(function(){ alert("The paragraph was clicked."); });
Equivalent JavaScript Code
document.addEventListener("click", function(eventArgs){ if (eventArgs.target.tagName.toLowerCase() === 'p') { alert("The paragraph was clicked."); } });
Website speed depends not just on what we have discussed here, this is just a small solution for the open-ended question, how we can increase the performance of the website.
Share this Article on
Tags: avoid jquery, javascript, website performance optimization,
RELATED POSTS